Deploy and Interact with Wrapped Token
Learn how to deploy and interact with wrapped tokens
In this section, we will deploy and interact with a wrapped token using Forge and cast commands. Forge simplifies smart contract deployment, and cast allows you to interact with deployed contracts.
1. Write the Wrapped Token Contract
Here is a basic implementation of a wrapped token contract in Solidity:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
contract WrappedToken is ERC20 {
address public nativeTokenHolder;
constructor() ERC20("Wrapped Token", "WTKN") {}
function deposit() external payable {
_mint(msg.sender, msg.value);
nativeTokenHolder = msg.sender;
}
function withdraw(uint256 amount) external {
_burn(msg.sender, amount);
payable(msg.sender).transfer(amount);
}
}
Deploy ERC20 Receiver
Deploy the Contract Using Forge's create
Command
forge create --rpc-url myblockchain --private-key $PK WrappedToken.sol:WrappedToken
Save the Wrapped Token Address
Save the Deployed to
address in an environment variable.
export WRAPPED_TOKEN=<address>
Interacting with the Deployed Contract
Once the contract is deployed, you can interact with it using cast commands.
You can use the following page for wei conversions.
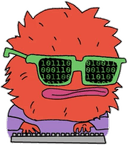
Time for a Quiz!
Wolfie wants to test your knowledge. Select the correct answer.
What is the primary purpose of wrapping a native token into an ERC-20 token?
ATo increase its supply
BTo burn the native token
CTo make the native token compatible with the ERC-20 standard
DTo mint more native tokens
Edit on GitHub
Last updated on 1/29/2025